//1 5개의 단어를 입력받아 배열에 저장한 후 출력하는 프로그램을 작성하시오. 각 단어의 길이는 20자 미만이다.
입력 예
jungol
information
gifted
center
higc
출력 예
jungol
information
gifted
center
higc
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
int i;
char strarr[5][20];
for (i = 0; i < 5; i++)
{
scanf("%s", strarr[i]);
}
for (i = 0; i < 5; i++)
{
printf("%s \n", strarr[i]);
}
return 0;
}
자가진단 1 5개의 단어를 입력받아 모든 단어를 입력받은 역순으로 출력하는 프로그램을 작성하시오. 각 단어의 길이는 30 이하이다.
입력 예
dog
cat
chick
calf
goat
출력 예
goat
calf
chick
cat
dog
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
int i;
char strarr[5][30];
for (i = 0; i < 5; i++)
{
scanf("%s", strarr[i]);
}
for (i = 4; i >= 0; i--)
{
printf("%s \n", strarr[i]);
}
return 0;
}
for (i = e; i < 5; i++) {
printf("%s strarr[4-i]
//2 49자 이하의 한 문장을 입력받아서 단어 단위로 구분하여 문자열을 배열에 저장하고 출력하는 프로그램을 작성하시오.
입력 예 I like you better than him.
출력 예
I
like
you
better
than
him.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, len;
int x = 0, y = 0;
char st[50];
char word[25][50];
fgets(st, 50, stdin);
len = strlen(st);
while (st[len - 1] == '\n' || st[len - 1] == '\r')
st[--len] = '0';
for (i = 0; i < len; i++)
{
if (st[i] == ' ')
{
word[x][y] = '\0';
x++;
y = 0;
}
else
{
word[x][y] = st[i];
y++;
}
}
word[x][y] = '\0';
x++;
for (i = 0; i < x; i++)
{
puts(word[i]);
}
return 0;
}
자가진단 2 공백을 포함한 문장을 입력받아서 홀수 번째 단어를 차례로 출력하는 프로그램을 작성하시오. 문장의 길이는 100자 이하이다.
입력 예 I like you better than him.
출력 예
I
you
than
(풀이1)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, len;
int x = 0, y = 0;
char st[50];
char word[25][50];
fgets(st, 50, stdin);
len = strlen(st);
while (st[len - 1] == '\n' || st[len - 1] == '\r')
st[--len] = '0';
for (i = 0; i < len; i++)
{
if (st[i] == ' ')
{
word[x][y] = '\0';
x++;
y = 0;
}
else
{
word[x][y] = st[i];
y++;
}
}
word[x][y] = '\0';
x++;
for (i = 0; i < x; i++)
{
if (i % 2 == 0)
{
puts(word[i]);
}
}
return 0;
}
(풀이2) : 이 방식이 더 간단함
for (i = 0; i < x; i += 2)
{
puts(word[i]);
}
//3 아래 소스와 같이 문자열 배열을 초기화하고, 문자 1개를 입력받아서 배열에 저장되어있는 단어들 중 입력받은 문자로 시작하는 단어를 모두 출력하는 프로그램을 작성하시오. 만약 없을 경우는 "찾는 단어가 없습니다." 라고 출력하시오.
입력 예 문자를 입력하세요. c
출력 예
champion
class
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, flag = 0;
char ch;
char word[10][20] = { "champion", "tel", "pencil", "olympiad", "class", "information", "jungol", "lesson", "book", "lion" };
printf("문자를 입력하세요. ");
scanf(" %c", &ch);
for (i = 0; i < 10; i++)
{
if (word[i][0] == ch)
{
puts(word[i]);
flag = 1;
}
}
if (flag == 0)
{
puts("찾는 단어가 없습니다.");
}
return 0;
}
자가진단 3 20개 이하의 문자로 이루어진 10개의 단어와 한 개의 문자를 입력받아서 마지막으로 입력받은 문자로 끝나는 단어를 모두 출력하는 프로그램을 작성하시오.
입력 예
champion
tel
pencil
olympiad
class
information
jungol
lesson
book
lion
l
출력 예
tel
pencil
jungol
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i;
char ch;
char word[10][20];
for (i = 0; i < 10; i++)
{
scanf("%s", word[i]);
}
scanf(" %c", &ch);
for (i = 0; i < 10; i++)
{
if (word[i][strlen(word[i]) - 1] == ch)
{
puts(word[i]);
}
}
return 0;
}
(풀이2)
for (i = 0; i < 10; i++)
{
len = strlen(word[i]);
if (word[i][len-1] == ch)
{
puts(word[i]);
}
}
//4 길이가 49 이하인 두 개의 문자열을 입력받아서 길이가 짧은 문자열을 먼저 출력하고 다음 줄에 나머지 문자열을 출력하는 프로그램을 작성하시오. (길이가 같은 경우에는 순서대로 출력한다.)
입력 예 information Jungol
출력 예
Jungol
Information
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[50], st2[50], tmp[50];
int len1, len2;
scanf("%s %s", st1, st2);
len1 = strlen(st1);
len2 = strlen(st2);
if (len1 > len2)
{
strcpy(tmp, st1);
strcpy(st1, st2);
strcpy(st2, tmp);
}
printf("%s \n%s \n", st1, st2);
return 0;
}
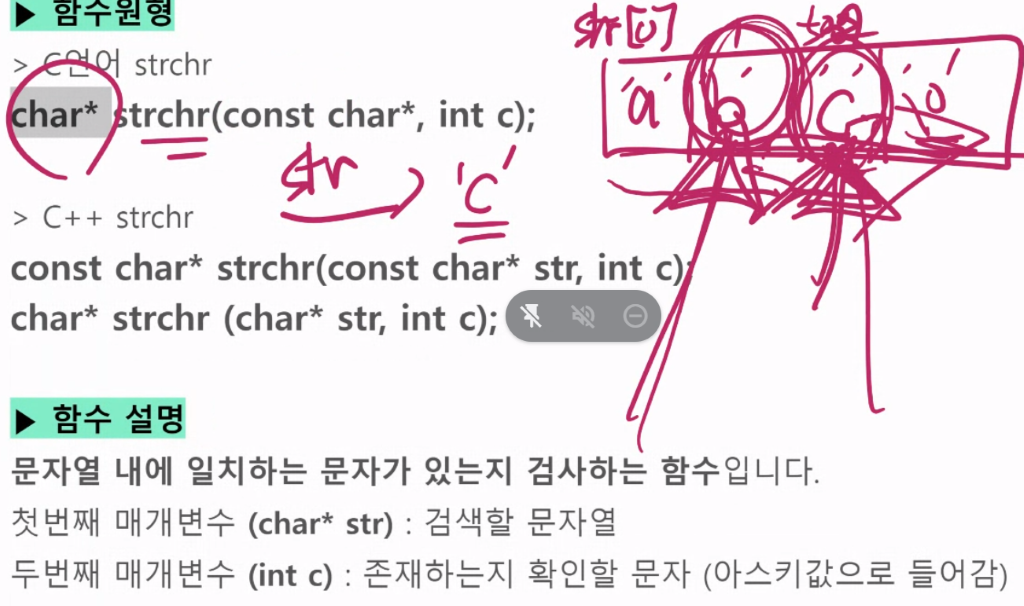
포인터 내부의 주소값 거기부터 찍어라 Null문자 만나기 전까지
자가진단 4 문자열을 선언하고 다음과 같이 "Hong Gil Dong"이라는 이름을 복사하여 저장한 후 출력하는 프로그램을 작성하시오.
출력 예 Hong Gil Dong
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st[50];
printf("%s", strcpy(st, "Hong Gil Dong"));
return 0;
}
(풀이1)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[50], st2[50];
int len;
fgets(st1, 50, stdin);
len = strlen(st1);
while (st1[len - 1] == '\n' || st1[len - 1] == '\r')
st1[--len] = '\0';
printf("%s", strcpy(st2, st1));
return 0;
}
(풀이2)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[50], st2[50];
scanf("%[^\n]", st1);
printf("%s", strcpy(st2, st1));
return 0;
}
//5 두 개의 문자열을 입력받아서 순서를 바꾸어 하나로 합치는 프로그램을 작성하시오.
입력 예 jungol olympiad
출력 예 olympiadjungol
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[50], st2[50], st3[100];
scanf("%s %s", st1, st2);
strcpy(st3, st2);
strcat(st3, st1);
puts(st3);
return 0;
}
자가진단 5 20개 이하의 문자열로 된 이름을 입력받아서 그 뒤에 "fighting"을 붙여서 저장하고 출력하는 프로그램을 작성하시오.
입력 예 Korea
출력 예 Koreafighting
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[20], st2[20] = "fighting";
scanf("%s", st1);
puts(strcat(st1, st2));
return 0;
}
//6 길이가 20 이하인 두 개의 문자열을 입력받아서 첫 번째 문자열의 앞부분 세 글자를 두 번째 문자열에 이어서 저장하고, 두 번째 문자열의 앞부분 세 글자를 첫 번째 문자열의 앞부분에 복사한 후 두 개의 문자열을 모두 출력하는 프로그램을 작성하시오.
입력 예 computer master
출력 예
masputer
mastercom
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[50], st2[50];
scanf("%s %s", st1, st2);
strncat(st2, st1, 3);
strncpy(st1, st2, 3);
puts(st1);
puts(st2);
return 0;
}
자가진단 6 20자 이하인 두 개의 문자열을 입력받아 첫 번째 문자열의 앞부분 두자를 두 번째 문자열의 앞부분에 복사하고 다시 뒷부분에 이어 붙여서 저장한 후 두 번째 문자열을 출력하는 프로그램을 작성하시오.
입력 예 ABCDE QWERTY
출력 예 ABERTYAB
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
char st1[20], st2[20];
scanf("%s %s", st1, st2);
strncpy(st2, st1, 2);
strncat(st2, st1, 2);
puts(st2);
return 0;
}
//7 문자열 배열을 {"champion", "tell", "pencil", "jungol", "olympiad", "class", "information", "lesson", "book", "lion"} 와 같이 초기화하고 문자 1개를 입력받아서 문자를 포함하고 있는 단어를 출력한 후, 다시 문자열 1개를 입력 받아 문자열을 포함하고 있는 단어를 찾아 출력하는 프로그램을 작성하시오. 만약 없으면 "찾는 단어가 없습니다." 라고 출력하시오.
입출력 예
문자를 입력하세요. I
champion
pencil
olympiad
information
lion
문자열을 입력하세요. pi
champion
olympiad
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, flag = 0;
char ch, st[20];
char word[10][20] = { "champion", "tel" , "pencil" , "jungol" , "olympiad" , "class" , "information" , "lesson" , "book" ,"lion" };
printf("문자를 입력하세요. ");
scanf(" %c", &ch);
for (i = 0; i < 10; i++)
{
if (strchr(word[i], ch))
{
puts(word[i]);
flag = 1;
}
}
if (flag == 0)
{
puts("찾는 단어가 없습니다.");
}
flag = 0;
printf("\n문자열을 입력하세요. ");
scanf("%s", st);
for (i = 0; i < 10; i++)
{
if (strstr(word[i], st))
{
puts(word[i]);
flag = 1;
}
}
if (flag == 0)
{
puts("찾는 단어가 없습니다.");
}
return 0;
}
자가진단 7 100개 이하의 문자로 구성된 한 개의 문자열을 입력받아서 그 문자열에 문자 'c'와 문자열 "ab"의 포함여부를 "Yes", "No"로 구분하여 출력하는 프로그램을 작성하시오.
입력 예 abdef
출력 예 No Yes
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, flag = 0;
char ch = 'c', st[3] = "ab";
char word[100];
scanf("%s", word);
if (strchr(word, ch))
{
printf("Yes ");
flag = 1;
}
if (flag == 0)
{
printf("No ");
}
flag = 0;
if (strstr(word, st))
{
printf("Yes ");
flag = 1;
}
if (flag == 0)
{
printf("No ");
}
return 0;
}
//8 20자 미만인 두 문자열을 입력받아 아스키코드를 기준으로 크기를 비교한 결과를 출력하고 앞의 세 문자가 같은지의 여부를 출력하는 프로그램을 작성하시오.
입력 예
jungol jungsu
japans korea
출력 예
jungsu 가(이) 더 큽니다.
앞의 세 문자가 같습니다.
Korea 가(이) 더 큽니다.
앞의 세 문자가 같지 않습니다.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int comp;
char st1[20], st2[20];
scanf("%s %s", st1, st2);
comp = strcmp(st1, st2);
if (comp == 0)
{
puts("두 문자열은 같습니다. \n");
}
else if (comp > 0)
{
printf("%s 가(이) 더 큽니다. \n", st1);
}
else
{
printf("%s 가(이) 더 큽니다. \n", st2);
}
if (strncmp(st1, st2, 3) == 0)
{
puts("앞의 세 문자가 같습니다.");
}
else
{
puts("앞의 세 문자가 같지 않습니다.");
}
return 0;
}
자가진단 8 20자 이하로 된 세 개의 단어를 입력받아 아스키코드 순으로 가장 적은 단어를 출력하는 프로그램을 작성하시오.
입력 예 cat dog cow
출력 예 cat
] int main()
char str1[21], str2[21], str3[21];
scanf "%s%s%s"
strl str2 str3
if (strcm (strl str2 < O && strcm (strl
printf( , strl);
else if {
str3
printf( ,
else {
printf( ,
return O;
str2);
str3);
(풀이 1)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int comp;
char st1[20], st2[20], st3[20], min[20];
scanf("%s %s %s", st1, st2, st3);
comp = strcmp(st1, st2);
if (comp <= 0)
{
strcpy(min, st1);
}
else
{
strcpy(min, st2);
}
comp = strcmp(min, st3);
if (comp <= 0)
{
strcpy(min, min);
}
else
{
strcpy(min, st3);
}
printf("%s", min);
return 0;
}
(풀이 2)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, j;
char word[3][20], result[20];
for (i = 0; i < 3; i++)
{
scanf("%s", word[i]);
}
for (i = 0; i < 2; i++)
{
for (j = i + 1; j < 3; j++)
{
if (strcmp(word[i], word[j]) < 0)
{
strcpy(result, word[i]);
}
}
}
printf("%s", result);
return 0;
}
//9 20자 미만인 5개의 단어를 입력 받아 문자열(아스키코드) 크기순으로 정렬하여 출력하는 프로그램을 작성하시오.
입력 예
단어 5개를 입력하세요.
Jungol
Korea
information
Monitor
class
출력 예
Jungol
Korea
Monitor
class
information
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, j;
char word[5][20], tmp[20];
puts("단어 5개를 입력하세요.");
for (i = 0; i < 5; i++)
{
scanf("%s", word[i]);
}
for (i = 0; i < 4; i++)
{
for (j = i + 1; j < 5; j++)
{
if (strcmp(word[i], word[j]) > 0)
{
strcpy(tmp, word[i]);
strcpy(word[i], word[j]);
strcpy(word[j], tmp);
}
}
}
for (i = 0; i < 5; i++)
{
puts(word[i]);
}
return 0;
}
자가진단 9 20자 미만인 5개의 문자열을 입력받아 문자열 크기(아스키코드) 역순으로 정렬하여 출력하는 프로그램을 작성하시오.
입력 예
Jungol
Korea
Information
Monitor
class
출력 예
information
class
Monitor
Korea
Jungol
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int i, j;
char word[5][20], tmp[20];
for (i = 0; i < 5; i++)
{
scanf("%s", word[i]);
}
for (i = 0; i < 4; i++)
{
for (j = i + 1; j < 5; j++)
{
if (strcmp(word[i], word[j]) < 0)
{
strcpy(tmp, word[i]);
strcpy(word[i], word[j]);
strcpy(word[j], tmp);
}
}
}
for (i = 0; i < 5; i++)
{
puts(word[i]);
}
return 0;
}
//10 정수형으로 된 두 개의 문자열을 입력받아 합계를 출력하고 실수형으로 된 두 개의 문자열을 입력받아 합계를 출력하는 프로그램을 작성하시오. (입력되는 문자열의 길이는 10자 이하이며 실수는 반올림하여 소수 둘째자리까지 출력한다. )
입력 예
123 4567
123.253 5236.12
출력 예
123 + 4567 = 4690
123.25 + 5236.12 = 5359.37
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int dnum1, dnum2, dhap;
double fnum1, fnum2;
char cnum1[11], cnum2[11];
scanf("%s %s", cnum1, cnum2);
dnum1 = atoi(cnum1);
dnum2 = atoi(cnum2);
dhap = dnum1 + dnum2;
printf("%d + %d = %d \n", dnum1, dnum2, dhap);
scanf("%s %s", cnum1, cnum2);
fnum1 = atof(cnum1);
fnum2 = atof(cnum2);
printf("%.2f + %.2f = %.2f \n", fnum1, fnum2, fnum1 + fnum2);
return 0;
}
자가진단 10 20자 미만인 한 개의 문자열을 입력받아서 첫 줄에는 정수로 변환하여 2배한 값을 출력하고, 다음 줄에는 실수로 변환한 값을 반올림하여 소수둘째자리까지 출력하는 프로그램을 작성하시오.
입력 예 50.1*34
출력 예
100
50.10
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
double fnum;
char word[20];
scanf("%s", word);
num = atoi(word);
fnum = atof(word);
printf("%d \n", num*2);
printf("%.2f \n", fnum);
return 0;
}
//11 3개의 실수를 입력받아서 각각을 소수 둘째자리까지 반올림하여 문자열 변수에 이어서 저장한 후 소수점('.')을 중심으로 행을 바꾸어 출력하는 프로그램을 작성하시오.
입력 예 123.456 78.90123 0.351
출력 예
123
4678
900
35
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
double a, b, c;
char str[200];
scanf("%lf %lf %lf", &a, &b, &c);
sprintf(str, "%.2f%.2f%.2f", a, b, c);
int i, len = strlen(str);
for (i = 0; i < len; i++)
{
if (str[i] == '.') printf("\n");
else printf("%c", str[i]);
}
return 0;
}
자가진단 11 5개의 정수를 입력받아 모두 붙여서 문자열로 저장한 후 세 자씩 나누어서 출력하는 프로그램을 작성하시오.
입력 예 12 5963 58 1 45678
출력 예
123
963
581
456
78
(풀이 1)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
int main()
{
int a, b, c, d, e;
char str[200], print[200];
scanf("%d %d %d %d %d", &a, &b, &c, &d, &e);
sprintf(str, "%d %d %d %d %d", a, b, c, d, e);
int i, j, len = strlen(str);
int cnt = 0;
for (i = 0; i < len; i++)
{
if (str[i] == ' ') i = i + 1;
if (cnt != 0 && cnt % 3 == 0) printf("\n");
printf("%c", str[i]);
cnt++;
}
return 0;
}
(풀이 2)
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main()
{
int a, b, c, d, e;
char str[200], print[200];
scanf("%d %d %d %d %d", &a, &b, &c, &d, &e);
sprintf(str, "%d %d %d %d %d", a, b, c, d, e);
int i, j, len = strlen(str);
int cnt = 0;
for (i = 0; i < len; i++)
{
if (isdigit(str[i]))
{
printf("%c", str[i]);
cnt++;
if (cnt != 0 && cnt % 3 == 0) printf("\n");
}
}
return 0;
}
'Basics > 자기주도 C언어 프로그래밍' 카테고리의 다른 글
[Chapter] 17 포인터 (0) | 2023.07.14 |
---|---|
[Chapter] 16 구조체 (0) | 2023.07.14 |
[Chapter] 14 문자열 I (0) | 2023.07.14 |
[Chapter] 13 함수 III (0) | 2023.07.14 |
[Chapter] 12 함수 II (0) | 2023.07.14 |